Koko loves to eat bananas. There are n piles of bananas, the ith pile has piles[i] bananas. The guards have gone and will come back in h hours.Koko can decide her bananas-per-hour eating speed of k. Each hour, she chooses some pile of bananas and eats k bananas from that pile. If the pile has less than k bananas, she eats all of them instead and will not eat any more bananas during this hour.Kok..
ํฐ์คํ ๋ฆฌ์ฑ๋ฆฐ์ง
You are given an m x n integer matrix matrix with the following two properties:Each row is sorted in non-decreasing order.The first integer of each row is greater than the last integer of the previous row.Given an integer target, return true if target is in matrix or false otherwise.You must write a solution in O(log(m * n)) time complexity.1. ๊ฐ ํ์ ์ค๋ฆ์ฐจ์์ผ๋ก ์ ๋ ฌ๋์ด ์์2. ํ ํ์ ์ฒซ ๋ฒ์งธ ๊ฐ์ ์ด์ ํ์ ๋ง์ง๋ง ๊ฐ๋ณด๋ค ํผO(..
You are given the heads of two sorted linked lists list1 and list2.Merge the two lists into one sorted list. The list should be made by splicing together the nodes of the first two lists.Return the head of the merged linked list.๋ ๊ฐ์ ๋งํฌ๋๋ฆฌ์คํธ๋ฅผ ํ๋์ sorted linked list๋ก ๋ง๋ค๊ธฐ! ํ์ด1: Recursion (์ฌ๊ท)# Definition for singly-linked list.# class ListNode:# def __init__(self, val=0, next=None):# se..
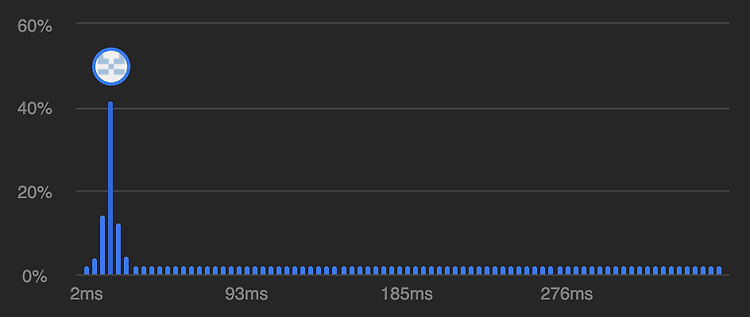
Given a string s, find the length of the longest substring without repeating characters.๋ฐ๋ณต๋๋ ๋ฌธ์ ์์ด ๊ฐ์ฅ ๊ธด substring์ ๊ธธ์ด๋ฅผ ์ฐพ๋ ๋ฌธ์ !substring์ ์์๊ณผ ๋์ด ์ ์๋ ์ ๋์ ์ธ ๋ฒ์์ด๋ฏ๋ก,์ํฉ์ ๋ฐ๋ผ ๋ฒ์(์๋์ฐ)๋ฅผ ๋๋ฆฌ๊ฑฐ๋ ์ค์ด๋ ์ฌ๋ผ์ด๋ฉ ์๋์ฐ ํ์ด๋ฅผ ๋ ์ฌ๋ฆด ์ ์๋ค. ํ์ด : Sliding Windowclass Solution: def lengthOfLongestSubstring(self, s: str) -> int: charSet = set() l = 0 res = 0 for r in range(len(s)): while s[..
Given head, the head of a linked list, determine if the linked list has a cycle in it.There is a cycle in a linked list if there is some node in the list that can be reached again by continuously following the next pointer. Internally, pos is used to denote the index of the node that tail's next pointer is connected to. Note that pos is not passed as a parameter.Return true if there is a cycle i..
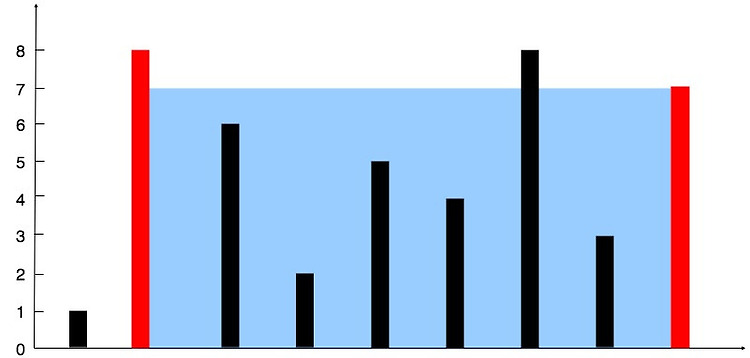
You are given an integer array height of length n. There are n vertical lines drawn such that the two endpoints of the ith line are (i, 0) and (i, height[i]).Find two lines that together with the x-axis form a container, such that the container contains the most water.Return the maximum amound of water a container can store.Notice that you may not slant the container.๊ทธ๋ฆผ์ ๋ณด๋ฉด ์ฝ๊ฒ ์ดํด๊ฐ ๊ฐ๋ฅํ๋ค. slant (๊ธฐ..
Given the head of a singly linked list, reverse the list, and return the reversed list.singly linked list ๊ฐ ์ฃผ์ด์ง๋ฉด, reversed list๋ฅผ ๋ฐํํ๋ ๋ฌธ์ ๋ค. ListNode ํด๋์คํ์ด์ฌ์์ ์ฐ๊ฒฐ๋ฆฌ์คํธ๋ฅผ ๊ตฌํํ๊ธฐ ์ํด์๋ ์ง์ ํด๋์ค๋ฅผ ์ ์ํด์ผ ํ๋ค.LeetCode ๋ฌธ์ ์์ ์ ๊ณต๋๋ ์ฐ๊ฒฐ ๋ฆฌ์คํธ ์ ์๋ ๋ค์๊ณผ ๊ฐ๋ค.# Definition for singly-linked list.# class ListNode:# def __init__(self, val=0, next=None):# self.val = val # ํ์ฌ ๋
ธ๋๊ฐ ์ ์ฅํ๋ ๊ฐ# self.next = next # ๋ค..
You are given an array prices where prices[i] is the price of a given stock on the ith day.You want to maximize your profit by choosing a single day to buy one stock and choosing a different day in the future to sell that stock.Return the maximum profit you can achieve from this transaction. If you cannot achieve any profit, return 0.์ฃผ์ ๊ฐ๊ฒฉ ๋ฐฐ์ด์ด ์ฃผ์ด์ก์ ๋, ์ป์ ์ ์๋ maximum profit์ ๋ฆฌํดํ๋ ๋ฌธ์ . ํ์ด1: ๋ธ๋ฃจํธํฌ์ค?..
Given an array of integers nums which is sorted in ascending order, and an integer target, write a function to search target in nums. If target exists, then return its index. Otherwise, return -1.You must write an algorithm with O(log n) runtime complexity.๊ฐ๋จํ ๋ฐ์ด๋๋ฆฌ์์น(์ด์งํ์) ๋ฌธ์ !์ด์งํ์์ ์ ๋ ฌ๋ ๋ฐฐ์ด์์๋ง ์ฌ์ฉํ ์ ์๋ ํ์ ์๊ณ ๋ฆฌ์ฆ์ด๋ค. ์ด์งํ์์ ๋งค๋ฒ ํ์ ๋ฒ์๋ฅผ ์ ๋ฐ์ผ๋ก ์ค์ด๋ฏ๋ก ์๊ฐ๋ณต์ก๋๊ฐ O(n)์ด๋ค.๋ํ ๋ฌธ์ ์์ ๋ช
์์ ์ผ๋ก O(log n) ์๊ฐ ๋ณต์ก๋๋ก ํด๊ฒฐํ๋ผ๊ณ ์๊ตฌํ๊ณ ์๋๋ฐ..
Given an array of integers temperatures represents the daily temperatures, return an array answer such that answer[i] is the number of days you have to wait after the ith day to get a warmer temperature. If there is no future day for which this is possible, keep answer[i] == 0 instead.์ฃผ์ด์ง ์จ๋ ๋ฐฐ์ด์์, ๊ฐ ๋ ์ดํ ๋ ๋ฐ๋ปํ ๋ ์ด ์ค๊ธฐ๊น์ง ๋ฉฐ์น ์ ๊ธฐ๋ค๋ ค์ผ ํ๋์ง ๊ณ์ฐํ๋ ๋ฌธ์ ์ด๋ค. ํ์ด1: ๋ธ๋ฃจํธ ํฌ์คclass Solution: def dailyTemperatures(s..